Configuration
Configuration details for initializing the Vinoshipper Injector.
The required Vinoshipper.init()
function takes up to two parameters.
Account ID
The Account ID is the required first parameter. This is a number made available to producers on the Vinoshipper platform and is located at Account -> Profile in the Vinoshipper Account login.
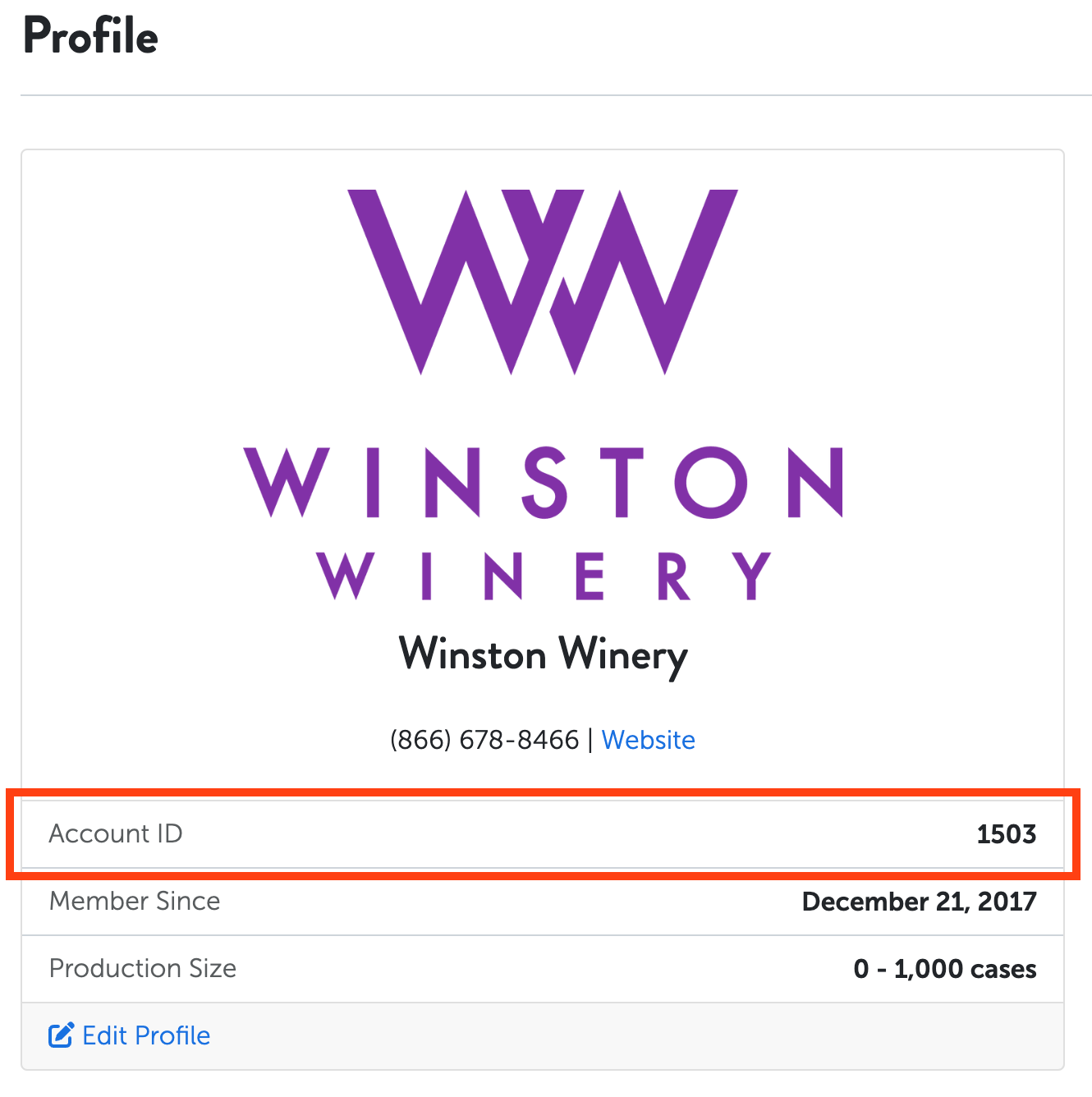
Sample display of Account -> Profile, highlighting the Account ID in a red box.
Configuration
Vinoshipper.init()
will accept an optional second parameter of an object of options. You will only need to include the specific settings you wish to change globally in your installation of Vinoshipper Injector. Options not defined will use the defaults.
The following example will set Vinoshipper Injector with the Account ID of 000
, the "Red" theme, and set the Cart Position to "start".
window.document.addEventListener('vinoshipper:loaded', () => {
window.Vinoshipper.init(000, {
theme: 'red',
cartPosition: 'start',
})
})
General Options
theme
theme
Type: string | null
Default: null
By default, the injector will use the “Blue” theming for all elements. This setting will change all elements to that theme style, unless overwritten by the HTML data-vs-theme
parameter of any specific instance.
For more information, see Simple Theming.
debug
debug
Type: boolean
Default: false
Enables additional logging to Vinoshipper Injector operations.
It is highly recommended to turn off debug when not actively developing.
skipAnalytics
skipAnalytics
Type: boolean
Default: false
Setting to true
will turn off analytics, regardless if supported analytics code is loaded onto the page.
Please see Analytics for more information.
autoRender
autoRender
Type: boolean
Default: true
When true
, Vinoshipper Injector will render all supported components on load and page load.
To manually trigger rendering, set this variable to false
and manually call Vinoshipper.render()
.
Vinoshipper will always render the Vinoshipper Cart. It will also render the Cart Button unless cartButton
is set to false
.
tooltips
tooltips
Type: boolean
Default: true
When true
, all Vinoshipper Injector components with tooltips on hover/focus will display. When false
, all tooltips will not display.
This can be overwritten per-element by using the HTML attribute data-vs-tooltip=""
with a truthy or falsy value.
Cart Options
Unlike other Vinoshipper components, the options for Vinoshipper Cart are all defined in the init()
function.
cartPosition
cartPosition
Default: 'end'
Options: 'start'
, 'end'
Displays the cart and cart button to either the start or end of the browser's window.
Start / End is the vocabulary similar to Flexbox start / end. For browsers set to left-tor-right languages, 'start'
is on the "left" and 'end'
is "right".
cartButton
cartButton
Type: boolean
Default: true
When false
, the standard Vinoshipper Cart Button will not render, allowing you to create your own cart button functionality.
You will need to implement your own cart button to allow the customer to show the cart. See Cart -> Methods for more information.
cartUrlParams
cartUrlParams
Type: Promise<{key: string, value: string}[]>
Default: undefined
If you are using a third-party analytics system that is not directly supported by Vinoshipper, this function will be fired every time the Checkout URL is needed. This allows you to append URL parameters for your Google Tag Manager implementation to support cross-domain tracking for your third-party analytics system.
We require a Promise
in order to support asynchronous callbacks. A successful return should be the form of an object of a key/string pair as follows:
window.document.addEventListener('vinoshipper:loaded', () => {
window.Vinoshipper.init(000, {
cartUrlParams: () => {
return yourParameterPromise()
.then((results) => {
returnKeyPairs = []
results.forEach((item) => {
returnKeyPairs.push({
key: item.key,
value: item.value
})
})
return returnKeyPairs
})
},
})
})
Static Data
If the values you wish to define are static, you must still define the return as a Promise.
window.document.addEventListener('vinoshipper:loaded', () => {
window.Vinoshipper.init(000, {
cartUrlParams: () => {
return Promise.resolve([
{
key: 'namedKey01',
value: '12345',
}
])
},
})
})
Example Data
Let's say the return object was this:
[
{
key: 'namedKey01',
value: 12345
},
{
key: 'namedKey01',
value: 12346
},
{
key: 'namedKey02',
value: 'a string'
]
The resulting checkout URL from the data above will be similar to this:
vinoshipper.com/cart?cartId=xxx-xxx&ret=http%3A%2F%2Fexample.com&namedKey01=12345&namedKey01=12346&namedKey02=a%20string
A few things to notice:
- All values are automatically converted to URL encoded strings.
namedKey01
appears twice as it's defined twice.cartId
andret
were added by Vinoshipper Injector.- If you have supported analytics enabled, Vinoshipper Injector will include these variables automatically.
Add-To-Cart Options
Advanced and globally set options for Add To Cart.
addToCartStyle
addToCartStyle
Type: Boolean | undefined
Default: true
When false
, style will NOT be included for all instances of Add To Cart. This allows you to add styles from a clean slate. However, you will be responsible for monitoring and adjusting to style changes to Vinoshipper Injector in the future.
If you plan to use Advanced Styling, do not define this setting.
Product Catalog Options
Advanced and globally set options for Product Catalog.
productCatalogAnnouncement
productCatalogAnnouncement
Type: Boolean | undefined
Default: true
When false
, all instances of Product Catalog will not include the Announcement component across all instances of Product Catalog. You will be responsible for including the component manually on your site.
productCatalogAvailable
productCatalogAvailable
Type: Boolean | undefined
Default: true
When false
, all instances of Product Catalog will not include the Available In component across all instances of Product Catalog. You will be responsible for including the component manually on your site.
productCatalogStyle
productCatalogStyle
Type: Boolean | undefined
Default: true
When false
, style will NOT be included for all instances of Product Catalog. This allows you to add styles from a clean slate. However, you will be responsible for monitoring and adjusting to style changes to Vinoshipper Injector in the future.
If you plan to use Advanced Styling, do not define this setting.
Announcement Options
Advanced and globally set options for Announcement.
announcementStyle
announcementStyle
Type: Boolean | undefined
Default: true
When false
, will not load Vinoshipper styles for the Announcement component. Useful when wanting to have full control over Announcement styling.
Available In Options
Advanced and globally set options for Available In.
availableInTooltips
availableInTooltips
Type: Boolean
Default: undefined
(reverts to tooltips
setting)
When true
, all Available In components, including inside Product Catalogs, will show tooltips on hover/focus will display. When false
, all tooltips will not display.
This can be overwritten per-element by using the HTML attribute data-vs-tooltip=""
with a truthy or falsy value.
Updated 5 months ago